Po przeczytaniu artykułu pt. "Open XML Format SDK 2.0 - pierwsze wrażenia", czytelnik wie już jak napisać prostą aplikację, która przy pomocy pakietu Open XML SDK i w języku C# tworzy prosty dokument typu Word 2007 (docx), który zawiera prosty tekst "Hello World!". Tym razem pójdziemy nieco dalej i dołożymy elementy związane z formatowaniem tekstu, czyli zajmiemy się stylami.
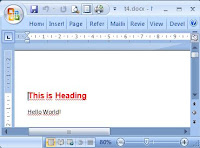
Załóżmy, że chcemy przygotować dokument, który będzie się składał z dwóch linijek tekstu. Pierwsza linijka będzie nagłówkiem akapitu, druga tekstem akapitu. To jak przygotować zwykły tekst (tak jak tutaj w drugiej linijce) pisałem o tym już wcześniej, tym razem napiszę jak przygotować tą pierwszą (odpowiednio sformatowaną) linijkę.
Pierwszą czynnością jaką należy wykonać, to trzeba dodać do tworzonego dokumentu część związaną z definicją stylów (dodajemy ją do głównego elementu dokumentu):
WordprocessingDocument myDoc = WordprocessingDocument.Create(documentFileName, WordprocessingDocumentType.Document) MainDocumentPart mainPart = myDoc.AddMainDocumentPart(); StyleDefinitionsPart stylePart = mainPart.AddNewPart<StyleDefinitionsPart>();
Definiujemy teraz czcionkę (Font), którą chcemy wykorzystać (w tym przykładzie: czerwony i pogrubiony Arial, rozmiaru 28):
// we have to set the properties RunProperties rPr = new RunProperties(); Color color = new Color() { Val = "FF0000" }; // the color is red RunFonts rFont = new RunFonts(); rFont.Ascii = "Arial"; // the font is Arial rPr.Append(color); rPr.Append(rFont); rPr.Append(new Bold()); // it is Bold rPr.Append(new FontSize() { Val = 28 }); //font size (in 1/72 of an inch)
Teraz czas na definicję stylu, będzie się on nazywać "My Heading 1", a jego identyfikator (używany później w dokumencie) to "MyHeading1". Oto definicja:
//creation of a style Style style = new Style(); style.StyleId = "MyHeading1"; //this is the ID of the style style.Append(new Name() { Val = "My Heading 1" }); //this is name style.Append(new BasedOn() { Val = "Heading1" }); // our style based on Normal style style.Append(new NextParagraphStyle() { Val = "Normal" }); // the next paragraph is Normal type style.Append(rPr);//we are adding properties previously defined
W tym momencie nadszedł czas na dodanie stworzonego stylu do dokumentu i zapisanie części związanej ze stylami:
// we have to add style that we have created to the StylePart stylePart.Styles = new Styles(); stylePart.Styles.Append(style); stylePart.Styles.Save(); // we save the style part
Styl mamy już stworzony, teraz trzeba go przypisać do jakiegoś paragrafu. Słyży do tego klasa ParagraphProperties, której trzeba przypisać odpowiednie ParagraphStyleId. Przykład poniżej:
Paragraph heading = new Paragraph(); Run heading_run = new Run(); Text heading_text = new Text("This is Heading"); ParagraphProperties heading_pPr = new ParagraphProperties(); heading_pPr.ParagraphStyleId = new ParagraphStyleId() { Val = "MyHeading1" }; // we set the style heading.Append(heading_pPr); heading_run.Append(heading_text); heading.Append(heading_run);
Teraz można już cieszyć się efektem naszych prac (przykład jest na załączonym rysunku), na sam koniec zamieszczę całą procedurę która generuje ten dokument:
private void HelloWorld_withStyle(string documentFileName) { // Create a Wordprocessing document. using (WordprocessingDocument myDoc = WordprocessingDocument.Create(documentFileName, WordprocessingDocumentType.Document)) { // Add a new main document part. MainDocumentPart mainPart = myDoc.AddMainDocumentPart(); //Add new style part StyleDefinitionsPart stylePart = mainPart.AddNewPart(); // we have to set the properties RunProperties rPr = new RunProperties(); Color color = new Color() { Val = "FF0000" }; // the color is red RunFonts rFont = new RunFonts(); rFont.Ascii = "Arial"; // the font is Arial rPr.Append(color); rPr.Append(rFont); rPr.Append(new Bold()); // it is Bold rPr.Append(new FontSize() { Val = 28 }); //font size (in 1/72 of an inch) //creation of a style Style style = new Style(); style.StyleId = "MyHeading1"; //this is the ID of the style style.Append(new Name() { Val = "My Heading 1" }); //this is name style.Append(new BasedOn() { Val = "Heading1" }); // our style based on Normal style style.Append(new NextParagraphStyle() { Val = "Normal" }); // the next paragraph is Normal type style.Append(rPr);//we are adding properties previously defined // we have to add style that we have created to the StylePart stylePart.Styles = new Styles(); stylePart.Styles.Append(style); stylePart.Styles.Save(); // we save the style part //Create Document tree for simple document. mainPart.Document = new Document(); //Create Body (this element contains other elements that we want to include Body body = new Body(); //Create paragraph Paragraph paragraph = new Paragraph(); Run run_paragraph = new Run(); // we want to put that text into the output document Text text_paragraph = new Text("Hello World!"); //Append elements appropriately. run_paragraph.Append(text_paragraph); paragraph.Append(run_paragraph); Paragraph heading = new Paragraph(); Run heading_run = new Run(); Text heading_text = new Text("This is Heading"); ParagraphProperties heading_pPr = new ParagraphProperties(); heading_pPr.ParagraphStyleId = new ParagraphStyleId() { Val = "MyHeading1" }; // we set the style heading.Append(heading_pPr); heading_run.Append(heading_text); heading.Append(heading_run); body.Append(heading); body.Append(paragraph); mainPart.Document.Append(body); // Save changes to the main document part. mainPart.Document.Save(); } }
Czytaj więcej tutaj: Tabele w Open XML Format SDK (DOCX) (czyli przygotowujemy tabliczkę mnożenia dla Workd 2007)
OdpowiedzUsuńJak wycentrowac taki nagłówek? ;-)
OdpowiedzUsuńJeśli chodzi o wyrównanie tekstu, to tutaj znajduje się post z odpowiedzią na postawione pytanie: http://maciej-progtech.blogspot.com/2009/08/na-lewo-na-prawo-hej-w-open-xml-pl.html
OdpowiedzUsuń